Lab 5 Instructions #
Reminders #
While other students are coming into class, follow the setup instructions below. (But please wait until you’re instructed before beginning work on the lab.)
If you still have some time before class, read the background on today’s lab puzzle below.
Preemptive high-five all your tablemates, in anticipation of a wonderful lab.
Swap drivers at each exercise. Nobody likes a driving hog.
We post lab solutions the next day, with extra information and ways of solving.
Setup instructions #
Head to http://url.cs51.io/lab5 and follow the Lab Procedures.
Lab puzzle #
Today’s puzzle, to be worked on individually, concerns a simple dice game called “doubles”, a variant of craps, which proceeds as follows: You roll two dice repeatedly until either you roll “doubles” (two of the same number) and lose or you roll a “natural” (in this game, a seven rolled as a three and a four) and win.
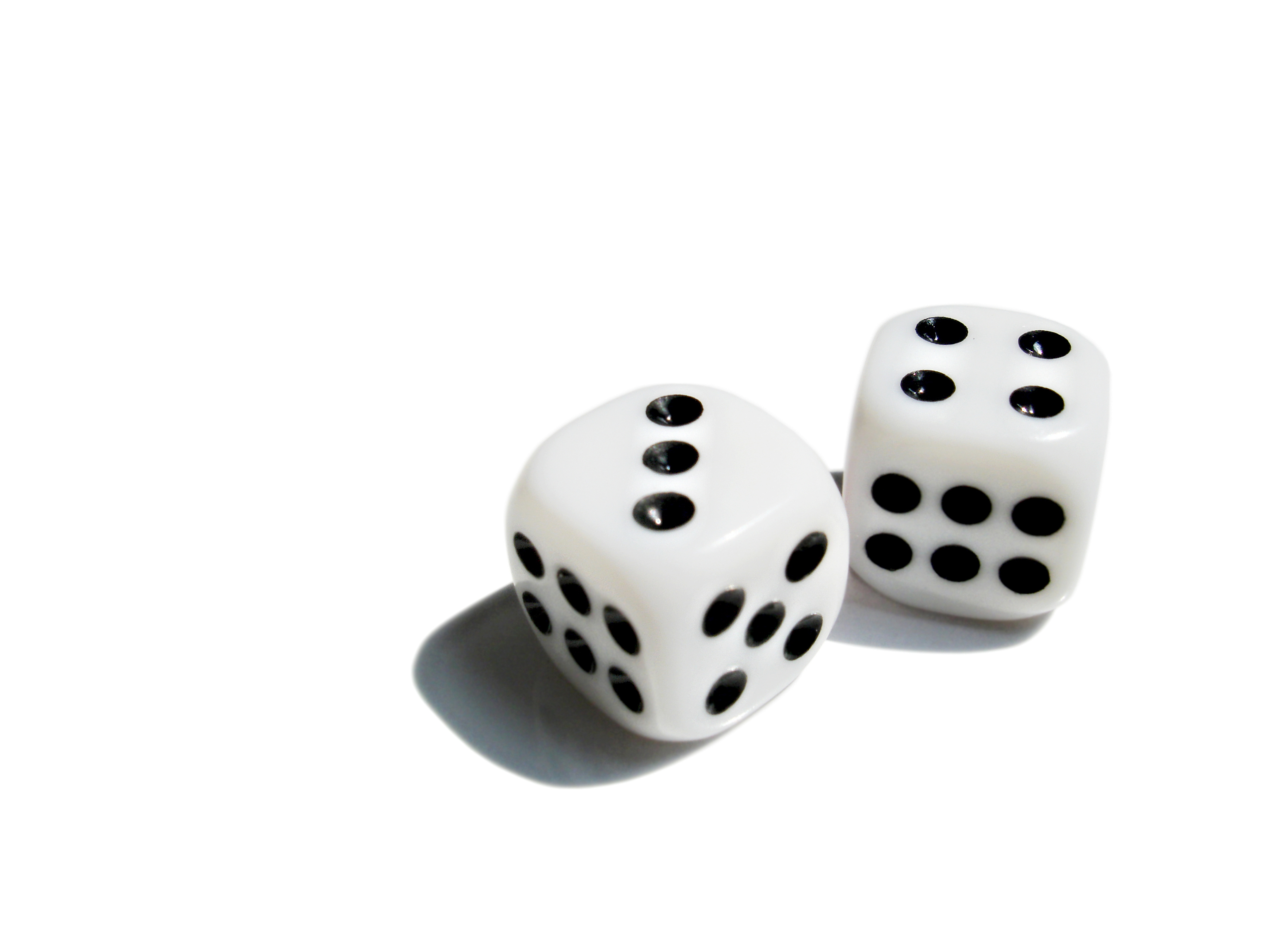
Figure 1: Rolling a “natural”
Appropriate types for the game and its outcomes are
type die =
One | Two | Three | Four | Five | Six ;;
type roll =
Roll of die * die ;;
type outcome =
| Doubles
| Natural
| Other ;;
Now we need a function that determines the outcome of a roll, either doubles, a natural, or something else. Below are some possible implementations of the function. Which ones work in the sense of generating no errors or warnings and having the correct behavior? (Please: No cheating by running the answers through OCaml. The idea is for you to figure it out yourself.)
A.
let outcome roll =
match roll with
| Roll (x, x) -> Doubles
| Roll (Three, Four)
| Roll (Four, Three) -> Natural
| _ -> Other ;;
B.
let outcome roll =
match roll with
| Roll (x, y) -> if x = y then Doubles else Other
| Roll (Three, Four)
| Roll (Four, Three) -> Natural
| _ -> Other ;;
C.
let outcome roll =
match roll with
| Roll (x, y) -> if x = y then Doubles
| Roll (Three, Four)
| Roll (Four, Three) -> Natural
| _ -> Other ;;
D.
let outcome roll =
match roll with
| Roll (Three, Four)
| Roll (Four, Three) -> Natural
| Roll (x, y) -> if x = y then Doubles else Other
| _ -> Other ;;
E.
let outcome roll =
match roll with
| Roll (Three, Four) -> Natural
| Roll (Four, Three) -> Natural
| Roll (x, x) -> Doubles
| _ -> Other ;;
F.
let outcome roll =
match roll with
| Roll (Three, Four)
| Roll (Four, Three) -> Natural
| Roll (x, y) -> if x = y then Doubles else Other
| Roll (_, _) -> Other ;;
G. None of them work.